Coding. It’s one of those things that seems daunting from a distance but becomes surprisingly manageable when you break it down. So, you're probably asking yourself, where do I even start? Well, let’s break it down into seven steps to make it all a bit less intimidating.
First up, understand the basics. It’s like learning the ABCs before writing a novel. Everything in coding builds on top of these fundamental ideas like algorithms, sequences, loops, and conditional statements. This foundational knowledge acts as your stepping stone to more complicated stuff down the road.
- Understanding the Basics
- Tools of the Trade
- Learning the Language
- Problem Solving Skills
- Writing Your First Code
- Debugging Essentials
- Keep Practicing
Understanding the Basics
The journey into coding starts with grasping some basic concepts. Think of it like learning to ride a bike. Before you hit those tricky mountain trails, you need to figure out the pedals and brakes. For coding, the fundamentals include algorithms, which are just step-by-step processes that computers follow to solve a problem or complete a task.
If you're scratching your head at the term algorithms, don’t stress. It's basically like a recipe: a set of instructions you give a computer to reach a desired outcome. Let's say you want a computer to tell you if a number is odd or even. The algorithm would involve a few steps: checking if the number is divisible by two and then returning 'even' or 'odd'. Simple, right?
Next up on the basics list are sequences. In coding, sequences are all about the order of operations. Like following the steps of a board game, coding needs specific steps executed in a particular order to work properly.
Loops and conditionals are two more key players. Loops allow you to repeat actions without writing the same lines of code over and over. Conditionals are your if-else statements, helping the program make decisions based on certain conditions. For example, if you're coding a game, you might have an if-condition that checks when the player hits an obstacle.
And let’s not forget data types like integers, strings, and booleans—think numbers, text, and true/false scenarios. These are part of your coder's toolkit for defining what kind of data your program will handle.
A little tip: aside from diving into tutorials, playing around with code in bite-sized projects can make these concepts stick. Try building a simple calculator or make a small game—anything that gets you familiar with these building blocks is a win!
Tools of the Trade
So, you've got your coding basics down, and you're ready to jump into the actual work. But wait, what tools do you need? Let’s chat about the essential gear you’ll want in your coding toolbox.
First things first, you’ll need a reliable computer. It doesn’t matter if it's a fancy laptop or a robust desktop; what matters is that it’s up to the task. You don’t want to be waiting around for a sluggish processor to catch up while your coding inspiration is flowing.
Next, pick a coding environment or IDE (Integrated Development Environment) that suits your style. Popular options include Visual Studio Code, PyCharm for Python lovers, or Atom, which is great for flexibility and has tons of extensions. Your IDE is your best friend during coding, so choose one that feels comfortable and supports your needs.
Don't forget about version control systems. Tools like Git allow you to track changes in your code, collaborate with others, and revert to previous versions if things go haywire. Knowing how to use Git is almost like having a safety net when trying out new ideas or collaborating on projects.
- Visual Studio Code: Great for most languages and very customizable.
- PyCharm: Ideal if you're diving into Python.
- Atom: Known for its flexibility and package ecosystem.
Whoa, we can't overlook online resources like Stack Overflow and GitHub. These platforms are gold mines for coders, full of community support, code snippets, and open-source projects. They keep your learning dynamic and help troubleshoot issues when you're stuck.
Lastly, there are debugging tools, which are crucial for smoothing out your code. Modern IDEs typically have built-in debuggers, but knowing the basics of debugging methods is invaluable. When your code doesn’t run as expected (and it will happen), debugging tools are your ace up your sleeve to hunt down and fix those pesky errors.
By arming yourself with these valuable tools, you're set up for a smoother coding journey. The right gear makes all the difference in how quickly you can excel and how much you enjoy the process. Remember, the better your tools, the more efficiently you’ll master coding steps and tackle any challenge that comes your way.
Learning the Language
Diving into coding is a lot like picking up a new language. Each has its own set of rules, grammar, and quirks. But here's the good news: you don't have to be a genius to understand it! The secret? Start with a language that suits your goals.
If you're interested in web development, HTML, CSS, and JavaScript are your bread and butter. They're pretty much everywhere online. Want to crunch numbers or analyze data? Python is your go-to. It's user-friendly and versatile, perfect for both beginners and seasoned pros.
Choosing to learn a specific coding language depends on what you want to achieve. Here's a little more detail:
- Python: Great for beginners, easy syntax, amazing for data science and web apps.
- JavaScript: Crucial for web development, works on both client- and server-side.
- Java: Popular in large systems development; think Android apps.
- C++: Offers control over system resources, needs good understanding of programming concepts.
- Ruby: Known for its elegant syntax, often used for web apps.
Whatever language you pick, focus on understanding its core. Don’t just memorize commands. Know why you use them. Online platforms like Codecademy or Khan Academy offer free, bite-sized lessons on these languages. You can even join community forums where you get to see real-life questions and how experts tackle them. This practical exposure is gold!
And remember, regular practice is key. Even if you spend just 20-30 minutes a day coding, you’ll start noticing how you slowly get fluent in this new language. The more you experiment, the more you learn. And don’t fret over mistakes—they’re just stepping stones to mastering coding.
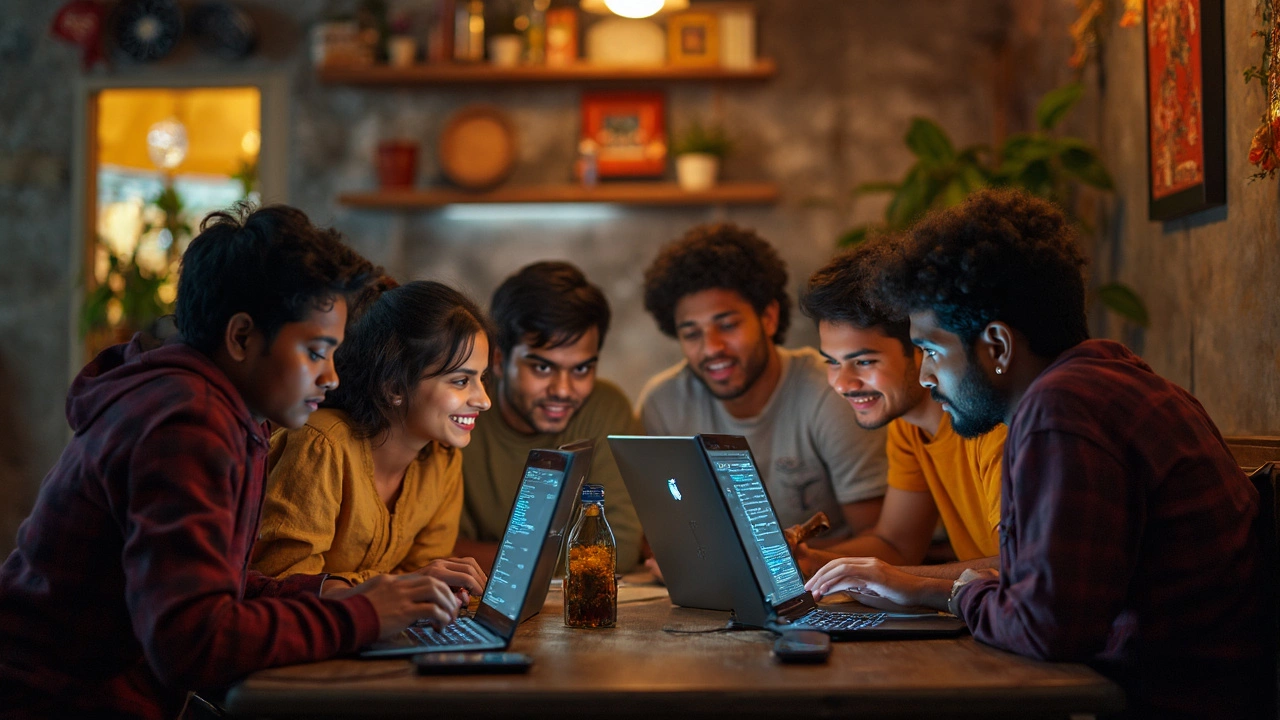
Problem Solving Skills
Alright, so you've got the basics down and know how to navigate your coding tools. Now comes one of the most exciting parts: developing strong problem-solving skills. Coding is pretty much all about solving puzzles, and sometimes those puzzles can be real brain busters!
Think of coding as being similar to putting together a piece of furniture from IKEA—but without the step-by-step instructions. You need to figure out which pieces go where and adapt if things don’t fit as expected. Here’s how you can sharpen those skills:
- Take it step-by-step: Break down the problem into smaller, more manageable parts. It helps to draw things out or use flowcharts to visualize the process. Understand that solving complex coding problems often starts with mastering these smaller bits.
- Think like a detective: Investigate every little detail. Why is this error occurring? What can data logs tell you? Start by examining the obvious before jumping into crazier theories.
- Embrace mistakes: Don’t fear errors; learn from them. Each mistake is an opportunity to understand your codes better. Debugging can be one of the most satisfying parts of coding when you finally solve a persistent issue.
You might be surprised to learn from a Stack Overflow survey that over 50% of developers say problem-solving is one of their favorite aspects of programming. So get comfortable putting on your detective hat—it’s a skill you’ll be using a lot!
If you want to really flex those problem-solving muscles, try coding challenges on platforms like LeetCode or Codewars. They provide real-world scenarios that help you think critically and work efficiently under pressure, which can be super helpful.
Writing Your First Code
Alright, you've grasped the basics, got your tools ready, and learned a bit of coding language. Now it's time to jump into the real action: writing your first code. This step is all about getting your hands dirty and understanding how things work by doing.
Remember when you first learned to ride a bike? You might have fallen a couple of times, but with each try, you got better. Coding is pretty similar. The best way to learn is by experimenting and playing around. Here’s a simple way to get started:
- Choose a simple project: Start with something straightforward, like printing 'Hello, World!' on your screen. This classic first project might seem trivial, but it teaches you how to write, compile, and execute code.
- Write the code: Open your coding environment, and type in your code. Here’s what it might look like in Python:
print("Hello, World!")
- Run the code: Hit that run button and watch the magic happen. Seeing the words 'Hello, World!' appear is oddly satisfying.
This initial success sets a strong foundation. But it doesn't stop there. As you grow more comfortable, gradually move on to more complex projects. You might build a simple calculator or a small shopping list app.
One fun fact: JavaScript, one of the most common coding languages, is used by 97.4% of websites as of 2023! It’s a great language to consider as you advance past the basics.
The key is to keep practicing and pushing your boundaries. Remember, every impressive coder started exactly where you are right now, trying to print 'Hello, World!'. Happy coding!
Debugging Essentials
So you've written your first line of code. Congratulations! But wait, it's not working? Welcome to coding! Debugging is a big part of the programming process, trust me, everyone runs into bugs, even the pros.
Debugging is like being a detective. You’ve got to identify what’s broken, figure out why it’s broken, and then fix it. Here are some steps you might find handy:
- Identify the Problem: Start by running your code to see the error message. Believe it or not, these messages are your friends. They point you to what's wrong and help you zero in on the problem.
- Check the Syntax: A lot of times it's something simple like a missing semicolon or a typo. Make sure everything is written correctly.
- Understand the Error Message: Take some time to read and understand what the error messages are saying. They might sound cryptic at first, but they’re packed with useful info.
- Use Print Statements: One quick way to check what your code is doing is by adding print statements at various points. You'll get to see which parts of your code aren’t acting the way they should.
A tip from my experience: stay calm. Debugging can be frustrating but it’s where you learn the most about coding. The more bugs you squash, the better you'll get at it.
Interestingly, about 50% of coding time can be spent debugging! It’s part of the game, and the more practice you get, the easier it becomes. Joy in coding success often comes from the satisfaction of solving these puzzles. So, next time you hit a bug, take a deep breath and remember, you're learning a vital skill.
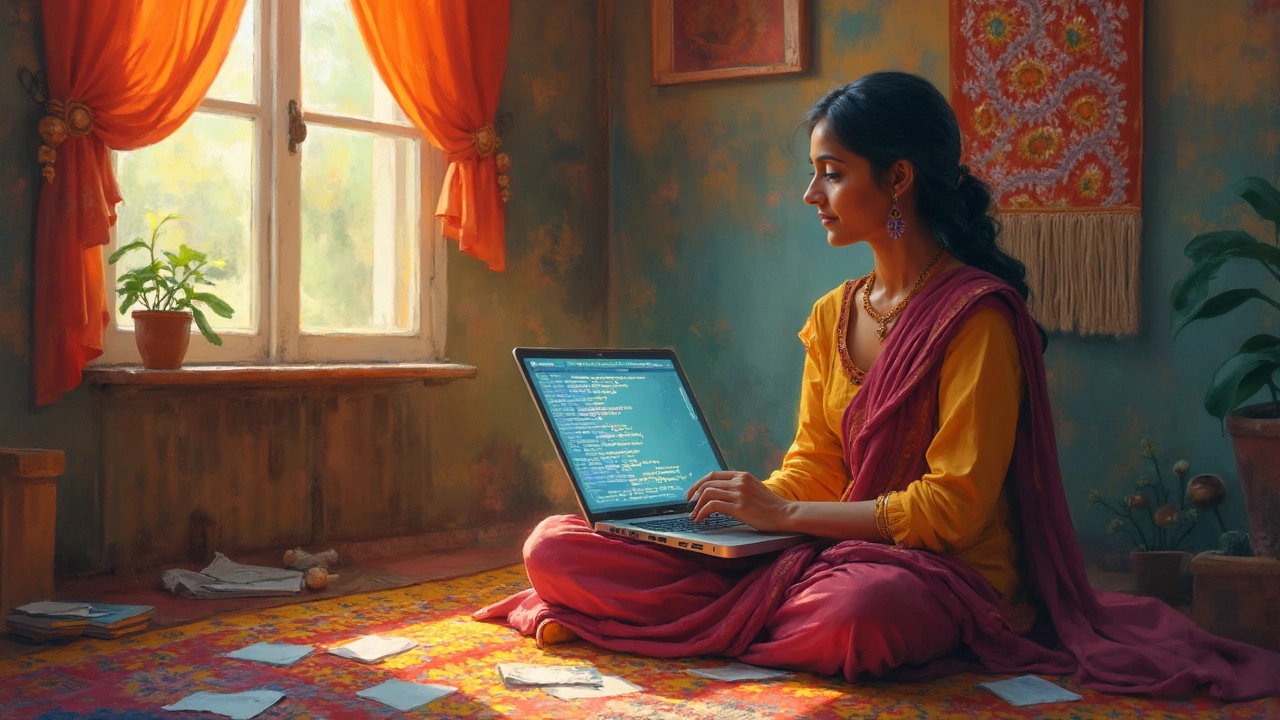
Keep Practicing
You know how they say practice makes perfect? Well, it's especially true with coding. You can't just read about coding steps and expect to ace it. Continuous practice helps you really get the hang of things and make fewer mistakes as you go.
Why is practice so crucial? Well, for starters, it helps reinforce what you've already learned. It's like learning to ride a bike. You didn't master it by watching others or reading a manual; you got on the bike and fell off a few times before you were cruising down the street. Coding is kind of the same way, just, you know, with less physical bruising.
Here's how you can ramp up your coding skills through consistent practice:
- Daily Challenges: Tackling small daily tasks or challenges is a good way to keep your skills sharp. Websites like HackerRank and LeetCode offer daily coding challenges that can push you further.
- Build Projects: Start small by creating little projects like a personal blog or a to-do list app. Building projects tests what you've learned and teaches you what to do when stuff doesn’t work out, which is just as important.
- Contribute to Open Source: This isn't just for experts. Beginners can contribute to smaller projects. It helps you see real-world coding in action and lets you collaborate with others—super useful!
Also, track your progress! Document what you did today versus last month or year. Seeing your growth can be a great motivator to continue practicing!